This is a SSCCE. Also posted in StackOverflow: https://stackoverflow.com/q/56500390/274677
I have a very simple Servlet that does nothing except print the value of a parameter:
public class TestServlet extends HttpServlet{
public void service(HttpServletRequest req, HttpServletResponse res) throws ServletException, IOException {
final String URL = req.getParameter("url");
System.out.printf("url parameter read as: [%s]\n", URL);
}
}
My application's web.xml is configured so as to automatically redirect http access to https:
<web-app>
...
<security-constraint>
<web-resource-collection>
<web-resource-name>SECURE</web-resource-name>
<url-pattern>/*</url-pattern>
</web-resource-collection>
<user-data-constraint>
<transport-guarantee>CONFIDENTIAL</transport-guarantee>
</user-data-constraint>
</security-constraint>
</web-app>
and I also have in my `standalone-full.xml` configuration file:
<http-listener name="default" socket-binding="http" redirect-socket="https" enable-http2="true"/>
If I deploy to JBoss EAP 7.1 and enter the following URL in my browser (where the `url` parameter carries the URL-encoded value of "http://www.google.com"):
http://localhost:8082/get-parameter-test/min?url=http%3A%2F%2Fwww.google.com
This is what I see in my developer console:
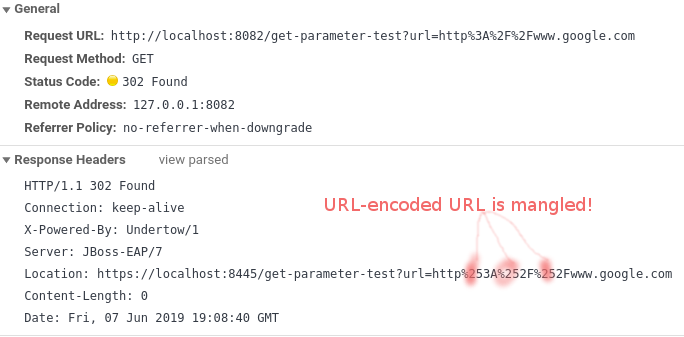
As a result after the automatic redirect, my code fails to obtain the correct value of the `url` parameter and I see on the log files:
url parameter read as: [http%3A%2F%2Fwww.google.com]
However, if I deploy to JBoss EAP 6.2 and do the same, the URL is not mangled in the redirect and everything works fine:
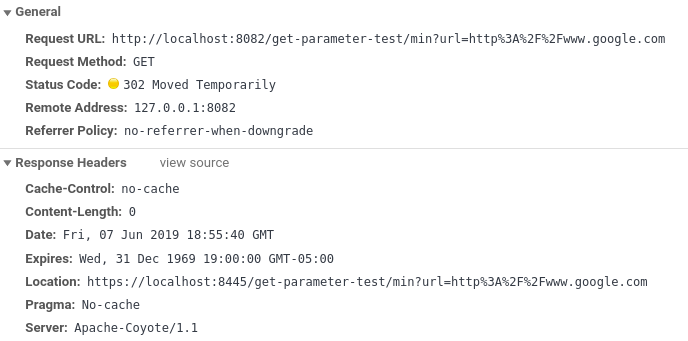