I generated server.jks and client.jks with keytool command and server- and client- properties files. I imported those files into META-INF folder. And I coded service endpoint interface, implementation class and callbackhandler class.
These are codes
===== IHelloWorld Interface
@WebService ( targetNamespace = "http://www.aaa.com/jbossws/ws-extensions/wssecurity" )
@PolicySets({"WS-Addressing","WS-SP-EX223_WSS11_Anonymous_X509_Sign_Encrypt"})
public interface IHelloWorld {
@WebMethod
@WebResult
public String sayHello(@WebParam String name);
}
=====HelloWorld.java
@WebService( portName = "HelloWorldServicePort",
serviceName = "HelloWorldService",
targetNamespace = "http://www.aaa.com/jbossws/ws-extensions/wssecurity",
endpointInterface = "com.aaa.ws.IHelloWorld" )
@EndpointConfig(configFile = "WEB-INF/jaxws-endpoint-config.xml", configName = "Custom WS-Security Endpoint") // this line DOES NOT WORK!!!
public class HelloWorld implements IHelloWorld {
@Override
public String sayHello(String name) {
// TODO Auto-generated method stub
return "Hello " + name;
}
}
====== KeystorePasswordCallback.java
public class KeystorePasswordCallback implements CallbackHandler {
private Map<String, String> passwords = new HashMap<String, String>();
public KeystorePasswordCallback() {
passwords.put("server", "password");
passwords.put("client", "password");
}
@Override
public void handle(Callback[] callbacks) throws IOException, UnsupportedCallbackException {
// TODO Auto-generated method stub
for (int i = 0; i < callbacks.length; i++) {
WSPasswordCallback pc = (WSPasswordCallback)callbacks[i];
String pass = passwords.get(pc.getIdentifier());
if (pass != null) {
pc.setPassword(pass);
return;
}
}
}
public void setAliasPassword(String alias, String password) {
passwords.put(alias, password);
}
}
===== jaxws-endpoint-config.xml
<jaxws-config xmlns="urn:jboss:jbossws-jaxws-config:4.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:javaee="http://java.sun.com/xml/ns/javaee"
xsi:schemaLocation="urn:jboss:jbossws-jaxws-config:4.0 schema/jbossws-jaxws-config_4_0.xsd">
<endpoint-config>
<config-name>Custom WS-Security Endpoint</config-name>
<property>
<property-name>ws-security.signature.properties</property-name>
<property-value>META-INF/server.properties</property-value>
</property>
<property>
<property-name>ws-security.encryption.properties</property-name>
<property-value>META-INF/server.properties</property-value>
</property>
<property>
<property-name>ws-security.signature.username</property-name>
<property-value>server</property-value>
</property>
<property>
<property-name>ws-security.encryption.username</property-name>
<property-value>client</property-value>
</property>
<property>
<property-name>ws-security.callback-handler</property-name>
<property-value>com.aaa.ws.KeystorePasswordCallback</property-value>
</property>
</endpoint-config>
</jaxws-config>
===== server.properties
org.apache.ws.security.crypto.provider=org.apache.ws.security.components.crypto.Merlin
org.apache.ws.security.crypto.merlin.keystore.type=jks
org.apache.ws.security.crypto.merlin.keystore.password=password
org.apache.ws.security.crypto.merlin.keystore.alias=server
org.apache.ws.security.crypto.merlin.keystore.file=META-INF/server.jks
===== client.properties
org.apache.ws.security.crypto.provider=org.apache.ws.security.components.crypto.Merlin
org.apache.ws.security.crypto.merlin.keystore.type=jks
org.apache.ws.security.crypto.merlin.keystore.password=password
org.apache.ws.security.crypto.merlin.keystore.alias=client
org.apache.ws.security.crypto.merlin.keystore.file=META-INF/client.jks
===== WSSClient.java
public class WSSClient {
private final String serviceURL="http://localhost:8080/WSSHelloWorld/HelloWorld";
private IHelloWorld proxy;
public WSSClient() throws IOException {
QName serviceName = new QName("http://www.aaa.com/jbossws/ws-extensions/wssecurity", "HelloWorldService");
URL wsdlURL = new URL(serviceURL + "?wsdl");
Service service = Service.create(wsdlURL, serviceName);
proxy = (IHelloWorld)service.getPort(IHelloWorld.class);
((BindingProvider)proxy).getRequestContext().put(SecurityConstants.CALLBACK_HANDLER, new KeystorePasswordCallback());
((BindingProvider)proxy).getRequestContext().put(SecurityConstants.SIGNATURE_PROPERTIES, Thread.currentThread().getContextClassLoader().getResource("META-INF/client.properties"));
((BindingProvider)proxy).getRequestContext().put(SecurityConstants.ENCRYPT_PROPERTIES, Thread.currentThread().getContextClassLoader().getResource("META-INF/client.properties"));
((BindingProvider)proxy).getRequestContext().put(SecurityConstants.SIGNATURE_USERNAME, "client");
((BindingProvider)proxy).getRequestContext().put(SecurityConstants.ENCRYPT_USERNAME, "server");
} // The constructor throws no exception. working well..
public String callMethd(String name) throws IOException {
return proxy.sayHello(name); // This line throws exception
}
}
===== index.jsp
<%@ page import="com.aaa.ws.*" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=EUC-KR">
<title>WS-Security Test</title>
</head>
<body>
<%
WSSClient client = new WSSClient();
out.println(client.callMethd("joseph")); // throws exception !!
%>
</body>
</html>
The following picture shows the folder structure and web service deployment process in eclipse ide.
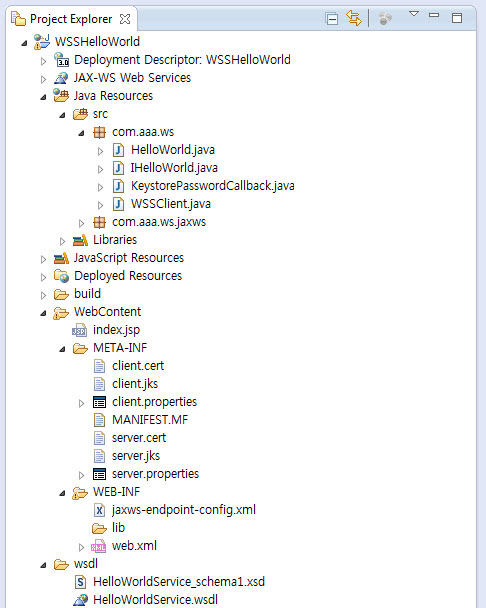
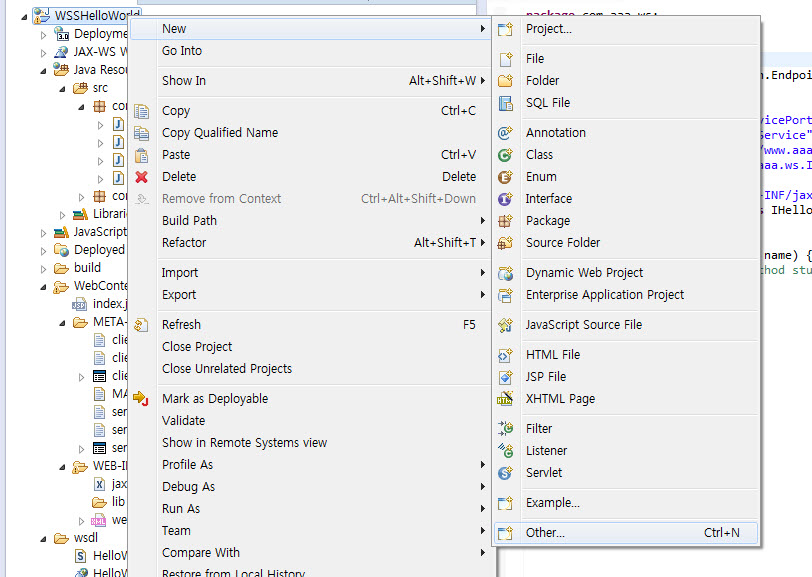
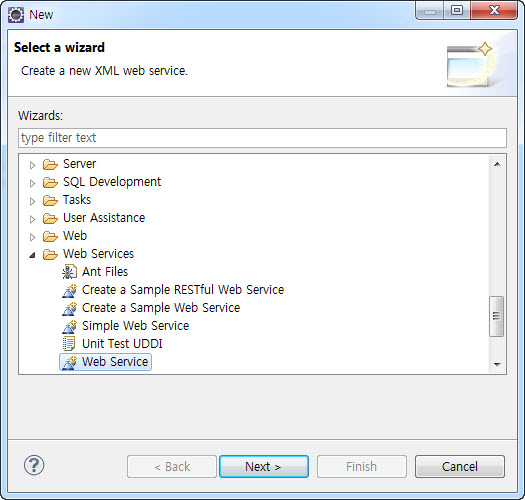
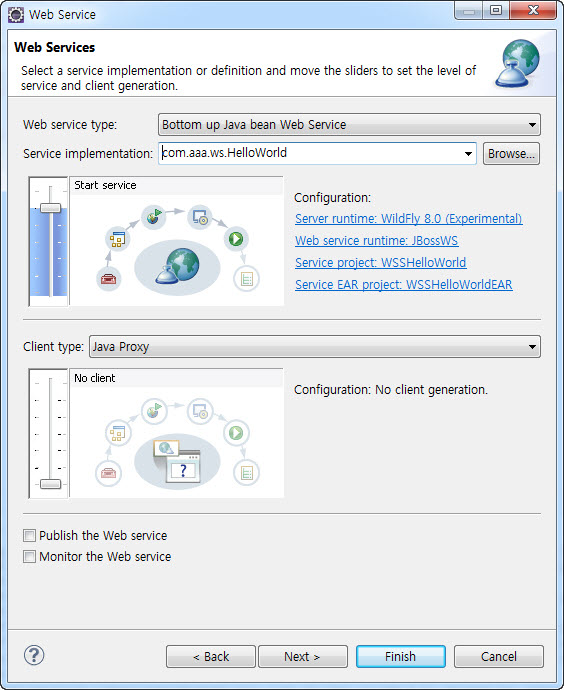
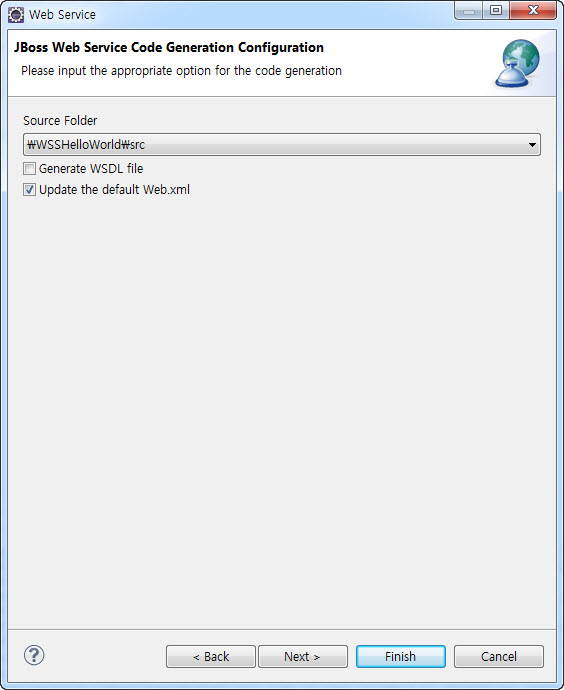
When index.jsp executed in WildFly 8.0 Alpha 4 server, it throws the exception like below,
19:30:21,337 WARNING [org.apache.cxf.phase.PhaseInterceptorChain] (default task-1) Interceptor for {http://www.aaa.com/jbossws/ws-extensions/wssecurity}HelloWorldService#{http://www.aaa.com/jbossws/ws-extensions/wssecurity}sayHello has thrown exception, unwinding now: org.apache.cxf.interceptor.Fault: Cannot encrypt data
at org.apache.cxf.ws.security.wss4j.policyhandlers.SymmetricBindingHandler.doSignBeforeEncrypt(SymmetricBindingHandler.java:395)
at org.apache.cxf.ws.security.wss4j.policyhandlers.SymmetricBindingHandler.handleBinding(SymmetricBindingHandler.java:124)
at org.apache.cxf.ws.security.wss4j.PolicyBasedWSS4JOutInterceptor$PolicyBasedWSS4JOutInterceptorInternal.handleMessage(PolicyBasedWSS4JOutInterceptor.java:166)
at org.apache.cxf.ws.security.wss4j.PolicyBasedWSS4JOutInterceptor$PolicyBasedWSS4JOutInterceptorInternal.handleMessage(PolicyBasedWSS4JOutInterceptor.java:90)
at org.apache.cxf.phase.PhaseInterceptorChain.doIntercept(PhaseInterceptorChain.java:271)
at org.apache.cxf.endpoint.ClientImpl.doInvoke(ClientImpl.java:541)
at org.apache.cxf.endpoint.ClientImpl.invoke(ClientImpl.java:474)
at org.apache.cxf.endpoint.ClientImpl.invoke(ClientImpl.java:377)
at org.apache.cxf.endpoint.ClientImpl.invoke(ClientImpl.java:330)
at org.apache.cxf.frontend.ClientProxy.invokeSync(ClientProxy.java:96)
at org.apache.cxf.jaxws.JaxWsClientProxy.invoke(JaxWsClientProxy.java:134)
at com.sun.proxy.$Proxy50.sayHello(Unknown Source)
at com.aaa.ws.WSSClient.callMethd(WSSClient.java:44) [classes:]
at org.apache.jsp.index_jsp._jspService(index_jsp.java:68)
at org.apache.jasper.runtime.HttpJspBase.service(HttpJspBase.java:69) [jastow-1.0.0.Beta1.jar:1.0.0.Beta1]
at javax.servlet.http.HttpServlet.service(HttpServlet.java:790) [jboss-servlet-api_3.1_spec-1.0.0.Beta1.jar:1.0.0.Beta1]
at org.apache.jasper.servlet.JspServletWrapper.service(JspServletWrapper.java:365) [jastow-1.0.0.Beta1.jar:1.0.0.Beta1]
at org.apache.jasper.servlet.JspServlet.serviceJspFile(JspServlet.java:321) [jastow-1.0.0.Beta1.jar:1.0.0.Beta1]
at org.apache.jasper.servlet.JspServlet.service(JspServlet.java:254) [jastow-1.0.0.Beta1.jar:1.0.0.Beta1]
at javax.servlet.http.HttpServlet.service(HttpServlet.java:790) [jboss-servlet-api_3.1_spec-1.0.0.Beta1.jar:1.0.0.Beta1]
at io.undertow.servlet.handlers.ServletHandler.handleRequest(ServletHandler.java:87) [undertow-servlet-1.0.0.Beta7.jar:1.0.0.Beta7]
at io.undertow.servlet.handlers.FilterHandler$FilterChainImpl.doFilter(FilterHandler.java:130) [undertow-servlet-1.0.0.Beta7.jar:1.0.0.Beta7]
at io.undertow.websockets.jsr.JsrWebSocketFilter.doFilter(JsrWebSocketFilter.java:136) [undertow-websockets-jsr-1.0.0.Beta7.jar:1.0.0.Beta7]
at io.undertow.servlet.core.ManagedFilter.doFilter(ManagedFilter.java:56) [undertow-servlet-1.0.0.Beta7.jar:1.0.0.Beta7]
at io.undertow.servlet.handlers.FilterHandler$FilterChainImpl.doFilter(FilterHandler.java:132) [undertow-servlet-1.0.0.Beta7.jar:1.0.0.Beta7]
at io.undertow.websockets.jsr.JsrWebSocketFilter.doFilter(JsrWebSocketFilter.java:136) [undertow-websockets-jsr-1.0.0.Beta7.jar:1.0.0.Beta7]
at io.undertow.servlet.core.ManagedFilter.doFilter(ManagedFilter.java:56) [undertow-servlet-1.0.0.Beta7.jar:1.0.0.Beta7]
at io.undertow.servlet.handlers.FilterHandler$FilterChainImpl.doFilter(FilterHandler.java:132) [undertow-servlet-1.0.0.Beta7.jar:1.0.0.Beta7]
at io.undertow.servlet.handlers.FilterHandler.handleRequest(FilterHandler.java:85) [undertow-servlet-1.0.0.Beta7.jar:1.0.0.Beta7]
at io.undertow.servlet.handlers.security.ServletSecurityRoleHandler.handleRequest(ServletSecurityRoleHandler.java:56) [undertow-servlet-1.0.0.Beta7.jar:1.0.0.Beta7]
at io.undertow.servlet.handlers.ServletDispatchingHandler.handleRequest(ServletDispatchingHandler.java:36) [undertow-servlet-1.0.0.Beta7.jar:1.0.0.Beta7]
at org.wildfly.extension.undertow.security.SecurityContextAssociationHandler.handleRequest(SecurityContextAssociationHandler.java:78)
at io.undertow.servlet.handlers.security.SSLInformationAssociationHandler.handleRequest(SSLInformationAssociationHandler.java:113) [undertow-servlet-1.0.0.Beta7.jar:1.0.0.Beta7]
at io.undertow.security.handlers.AuthenticationCallHandler.handleRequest(AuthenticationCallHandler.java:52) [undertow-core-1.0.0.Beta7.jar:1.0.0.Beta7]
at io.undertow.security.handlers.AbstractConfidentialityHandler.handleRequest(AbstractConfidentialityHandler.java:45) [undertow-core-1.0.0.Beta7.jar:1.0.0.Beta7]
at io.undertow.servlet.handlers.security.CachedAuthenticatedSessionHandler.handleRequest(CachedAuthenticatedSessionHandler.java:65) [undertow-servlet-1.0.0.Beta7.jar:1.0.0.Beta7]
at io.undertow.security.handlers.SecurityInitialHandler.handleRequest(SecurityInitialHandler.java:70) [undertow-core-1.0.0.Beta7.jar:1.0.0.Beta7]
at io.undertow.server.handlers.PredicateHandler.handleRequest(PredicateHandler.java:25) [undertow-core-1.0.0.Beta7.jar:1.0.0.Beta7]
at org.wildfly.extension.undertow.security.SecurityContextCreationHandler.handleRequest(SecurityContextCreationHandler.java:54)
at io.undertow.server.handlers.PredicateHandler.handleRequest(PredicateHandler.java:25) [undertow-core-1.0.0.Beta7.jar:1.0.0.Beta7]
at io.undertow.servlet.handlers.ServletInitialHandler.handleFirstRequest(ServletInitialHandler.java:207) [undertow-servlet-1.0.0.Beta7.jar:1.0.0.Beta7]
at io.undertow.servlet.handlers.ServletInitialHandler.dispatchRequest(ServletInitialHandler.java:194) [undertow-servlet-1.0.0.Beta7.jar:1.0.0.Beta7]
at io.undertow.servlet.handlers.ServletInitialHandler.access$000(ServletInitialHandler.java:72) [undertow-servlet-1.0.0.Beta7.jar:1.0.0.Beta7]
at io.undertow.servlet.handlers.ServletInitialHandler$1.handleRequest(ServletInitialHandler.java:128) [undertow-servlet-1.0.0.Beta7.jar:1.0.0.Beta7]
at io.undertow.server.HttpHandlers.executeRootHandler(HttpHandlers.java:36) [undertow-core-1.0.0.Beta7.jar:1.0.0.Beta7]
at io.undertow.server.HttpServerExchange$1.run(HttpServerExchange.java:628) [undertow-core-1.0.0.Beta7.jar:1.0.0.Beta7]
at java.util.concurrent.ThreadPoolExecutor.runWorker(Unknown Source) [rt.jar:1.7.0_25]
at java.util.concurrent.ThreadPoolExecutor$Worker.run(Unknown Source) [rt.jar:1.7.0_25]
at java.lang.Thread.run(Unknown Source) [rt.jar:1.7.0_25]
Caused by: org.apache.cxf.ws.policy.PolicyException: Cannot encrypt data
at org.apache.cxf.ws.security.wss4j.policyhandlers.AbstractBindingBuilder.policyNotAsserted(AbstractBindingBuilder.java:294)
at org.apache.cxf.ws.security.wss4j.policyhandlers.SymmetricBindingHandler.doEncryptionDerived(SymmetricBindingHandler.java:497)
at org.apache.cxf.ws.security.wss4j.policyhandlers.SymmetricBindingHandler.doEncryption(SymmetricBindingHandler.java:514)
at org.apache.cxf.ws.security.wss4j.policyhandlers.SymmetricBindingHandler.doSignBeforeEncrypt(SymmetricBindingHandler.java:389)
... 48 more
19:30:21,345 ERROR [io.undertow.request] (default task-1) Servlet request failed HttpServerExchange{ GET /WSSHelloWorld/index.jsp}: org.apache.jasper.JasperException: javax.xml.ws.soap.SOAPFaultException: Cannot encrypt data
at org.apache.jasper.servlet.JspServletWrapper.service(JspServletWrapper.java:409) [jastow-1.0.0.Beta1.jar:1.0.0.Beta1]
at org.apache.jasper.servlet.JspServlet.serviceJspFile(JspServlet.java:321) [jastow-1.0.0.Beta1.jar:1.0.0.Beta1]
at org.apache.jasper.servlet.JspServlet.service(JspServlet.java:254) [jastow-1.0.0.Beta1.jar:1.0.0.Beta1]
at javax.servlet.http.HttpServlet.service(HttpServlet.java:790) [jboss-servlet-api_3.1_spec-1.0.0.Beta1.jar:1.0.0.Beta1]
at io.undertow.servlet.handlers.ServletHandler.handleRequest(ServletHandler.java:87) [undertow-servlet-1.0.0.Beta7.jar:1.0.0.Beta7]
at io.undertow.servlet.handlers.FilterHandler$FilterChainImpl.doFilter(FilterHandler.java:130) [undertow-servlet-1.0.0.Beta7.jar:1.0.0.Beta7]
at io.undertow.websockets.jsr.JsrWebSocketFilter.doFilter(JsrWebSocketFilter.java:136) [undertow-websockets-jsr-1.0.0.Beta7.jar:1.0.0.Beta7]
at io.undertow.servlet.core.ManagedFilter.doFilter(ManagedFilter.java:56) [undertow-servlet-1.0.0.Beta7.jar:1.0.0.Beta7]
at io.undertow.servlet.handlers.FilterHandler$FilterChainImpl.doFilter(FilterHandler.java:132) [undertow-servlet-1.0.0.Beta7.jar:1.0.0.Beta7]
at io.undertow.websockets.jsr.JsrWebSocketFilter.doFilter(JsrWebSocketFilter.java:136) [undertow-websockets-jsr-1.0.0.Beta7.jar:1.0.0.Beta7]
at io.undertow.servlet.core.ManagedFilter.doFilter(ManagedFilter.java:56) [undertow-servlet-1.0.0.Beta7.jar:1.0.0.Beta7]
at io.undertow.servlet.handlers.FilterHandler$FilterChainImpl.doFilter(FilterHandler.java:132) [undertow-servlet-1.0.0.Beta7.jar:1.0.0.Beta7]
at io.undertow.servlet.handlers.FilterHandler.handleRequest(FilterHandler.java:85) [undertow-servlet-1.0.0.Beta7.jar:1.0.0.Beta7]
at io.undertow.servlet.handlers.security.ServletSecurityRoleHandler.handleRequest(ServletSecurityRoleHandler.java:56) [undertow-servlet-1.0.0.Beta7.jar:1.0.0.Beta7]
at io.undertow.servlet.handlers.ServletDispatchingHandler.handleRequest(ServletDispatchingHandler.java:36) [undertow-servlet-1.0.0.Beta7.jar:1.0.0.Beta7]
at org.wildfly.extension.undertow.security.SecurityContextAssociationHandler.handleRequest(SecurityContextAssociationHandler.java:78)
at io.undertow.servlet.handlers.security.SSLInformationAssociationHandler.handleRequest(SSLInformationAssociationHandler.java:113) [undertow-servlet-1.0.0.Beta7.jar:1.0.0.Beta7]
at io.undertow.security.handlers.AuthenticationCallHandler.handleRequest(AuthenticationCallHandler.java:52) [undertow-core-1.0.0.Beta7.jar:1.0.0.Beta7]
at io.undertow.security.handlers.AbstractConfidentialityHandler.handleRequest(AbstractConfidentialityHandler.java:45) [undertow-core-1.0.0.Beta7.jar:1.0.0.Beta7]
at io.undertow.servlet.handlers.security.CachedAuthenticatedSessionHandler.handleRequest(CachedAuthenticatedSessionHandler.java:65) [undertow-servlet-1.0.0.Beta7.jar:1.0.0.Beta7]
at io.undertow.security.handlers.SecurityInitialHandler.handleRequest(SecurityInitialHandler.java:70) [undertow-core-1.0.0.Beta7.jar:1.0.0.Beta7]
at io.undertow.server.handlers.PredicateHandler.handleRequest(PredicateHandler.java:25) [undertow-core-1.0.0.Beta7.jar:1.0.0.Beta7]
at org.wildfly.extension.undertow.security.SecurityContextCreationHandler.handleRequest(SecurityContextCreationHandler.java:54)
at io.undertow.server.handlers.PredicateHandler.handleRequest(PredicateHandler.java:25) [undertow-core-1.0.0.Beta7.jar:1.0.0.Beta7]
at io.undertow.servlet.handlers.ServletInitialHandler.handleFirstRequest(ServletInitialHandler.java:207) [undertow-servlet-1.0.0.Beta7.jar:1.0.0.Beta7]
at io.undertow.servlet.handlers.ServletInitialHandler.dispatchRequest(ServletInitialHandler.java:194) [undertow-servlet-1.0.0.Beta7.jar:1.0.0.Beta7]
at io.undertow.servlet.handlers.ServletInitialHandler.access$000(ServletInitialHandler.java:72) [undertow-servlet-1.0.0.Beta7.jar:1.0.0.Beta7]
at io.undertow.servlet.handlers.ServletInitialHandler$1.handleRequest(ServletInitialHandler.java:128) [undertow-servlet-1.0.0.Beta7.jar:1.0.0.Beta7]
at io.undertow.server.HttpHandlers.executeRootHandler(HttpHandlers.java:36) [undertow-core-1.0.0.Beta7.jar:1.0.0.Beta7]
at io.undertow.server.HttpServerExchange$1.run(HttpServerExchange.java:628) [undertow-core-1.0.0.Beta7.jar:1.0.0.Beta7]
at java.util.concurrent.ThreadPoolExecutor.runWorker(Unknown Source) [rt.jar:1.7.0_25]
at java.util.concurrent.ThreadPoolExecutor$Worker.run(Unknown Source) [rt.jar:1.7.0_25]
at java.lang.Thread.run(Unknown Source) [rt.jar:1.7.0_25]
Caused by: javax.xml.ws.soap.SOAPFaultException: Cannot encrypt data
at org.apache.cxf.jaxws.JaxWsClientProxy.invoke(JaxWsClientProxy.java:156)
at com.sun.proxy.$Proxy50.sayHello(Unknown Source)
at com.aaa.ws.WSSClient.callMethd(WSSClient.java:44) [classes:]
at org.apache.jsp.index_jsp._jspService(index_jsp.java:68)
at org.apache.jasper.runtime.HttpJspBase.service(HttpJspBase.java:69) [jastow-1.0.0.Beta1.jar:1.0.0.Beta1]
at javax.servlet.http.HttpServlet.service(HttpServlet.java:790) [jboss-servlet-api_3.1_spec-1.0.0.Beta1.jar:1.0.0.Beta1]
at org.apache.jasper.servlet.JspServletWrapper.service(JspServletWrapper.java:365) [jastow-1.0.0.Beta1.jar:1.0.0.Beta1]
... 32 more
Caused by: org.apache.cxf.ws.policy.PolicyException: Cannot encrypt data
at org.apache.cxf.ws.security.wss4j.policyhandlers.AbstractBindingBuilder.policyNotAsserted(AbstractBindingBuilder.java:294)
at org.apache.cxf.ws.security.wss4j.policyhandlers.SymmetricBindingHandler.doEncryptionDerived(SymmetricBindingHandler.java:497)
at org.apache.cxf.ws.security.wss4j.policyhandlers.SymmetricBindingHandler.doEncryption(SymmetricBindingHandler.java:514)
at org.apache.cxf.ws.security.wss4j.policyhandlers.SymmetricBindingHandler.doSignBeforeEncrypt(SymmetricBindingHandler.java:389)
at org.apache.cxf.ws.security.wss4j.policyhandlers.SymmetricBindingHandler.handleBinding(SymmetricBindingHandler.java:124)
at org.apache.cxf.ws.security.wss4j.PolicyBasedWSS4JOutInterceptor$PolicyBasedWSS4JOutInterceptorInternal.handleMessage(PolicyBasedWSS4JOutInterceptor.java:166)
at org.apache.cxf.ws.security.wss4j.PolicyBasedWSS4JOutInterceptor$PolicyBasedWSS4JOutInterceptorInternal.handleMessage(PolicyBasedWSS4JOutInterceptor.java:90)
at org.apache.cxf.phase.PhaseInterceptorChain.doIntercept(PhaseInterceptorChain.java:271)
at org.apache.cxf.endpoint.ClientImpl.doInvoke(ClientImpl.java:541)
at org.apache.cxf.endpoint.ClientImpl.invoke(ClientImpl.java:474)
at org.apache.cxf.endpoint.ClientImpl.invoke(ClientImpl.java:377)
at org.apache.cxf.endpoint.ClientImpl.invoke(ClientImpl.java:330)
at org.apache.cxf.frontend.ClientProxy.invokeSync(ClientProxy.java:96)
at org.apache.cxf.jaxws.JaxWsClientProxy.invoke(JaxWsClientProxy.java:134)